29 August 2024
Feature flags are a powerful asset in software development, allowing you to toggle features on or off without deploying new code. This flexibility serves various purposes, including:
- A/B Testing: Run A/B tests on new features to gauge user reactions before a full rollout.
- Incremental Rollouts: Gradually introduce new features to a subset of users before expanding availability.
- Environment-Specific Controls: Manage feature access across different environments like development, staging, and production.
The Unleash SDK for React streamlines the process of incorporating feature flags into your React applications with the following benefits:
- Simple Declarative Syntax: Define feature flags using a straightforward, declarative approach.
- Optimized Rendering: Enhance performance by deferring rendering until feature flags are fetched.
- Class and Functional Component Compatibility: Support for both class-based and functional components.
Installation
1. Setting up a self-hosted Unleash server: The easiest way to run Unleash locally is by using Git and Docker.
git clone [email protected]:Unleash/unleash.git
cd unleash
docker compose up -d
2. Accessing the Unleash GUI: Once Docker is up and running, navigate to http://localhost:4242/. You’ll see a login screen.
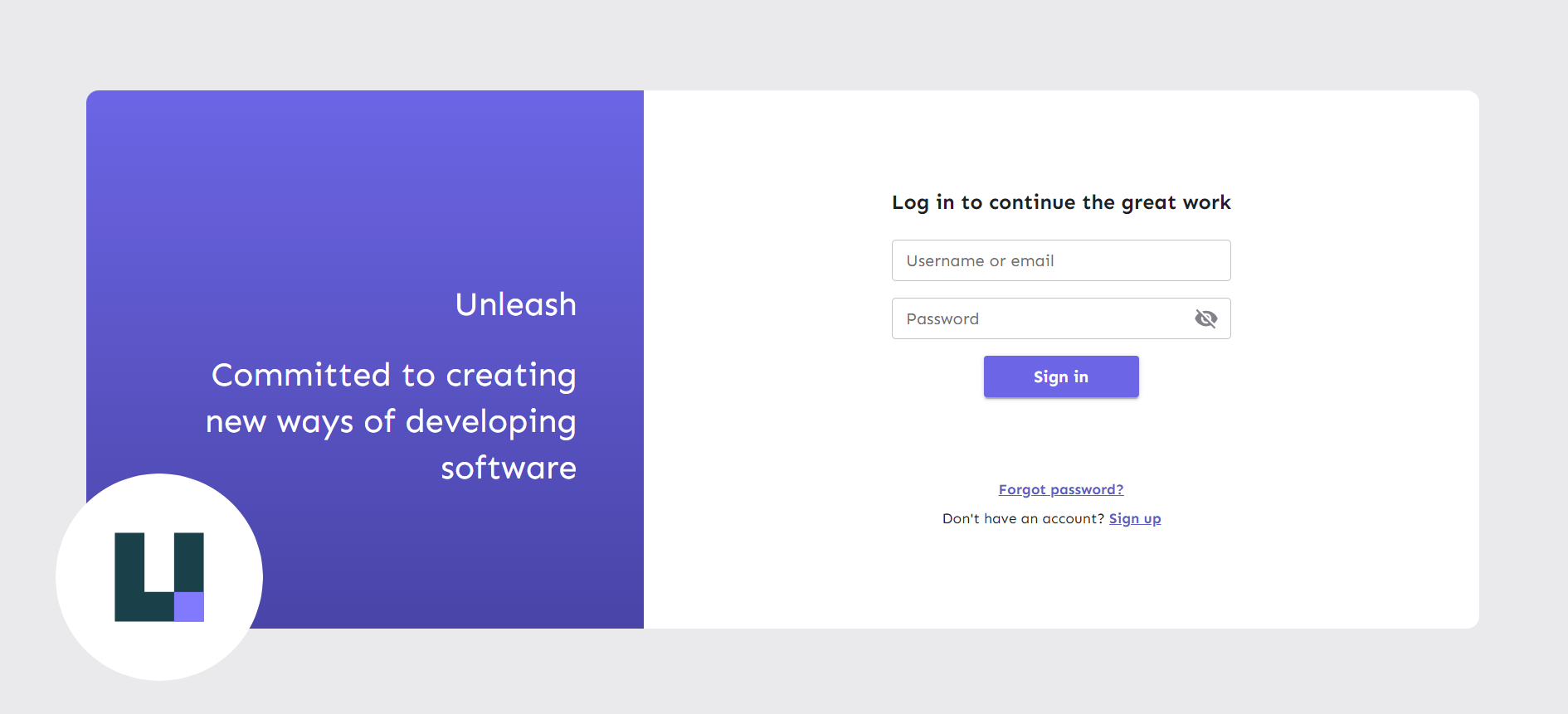
Use the following credentials to login:
username: admin
password: unleash4all
Creating an API access token
Go to the “API Access” tab in the side navigation and click “New API Token.”
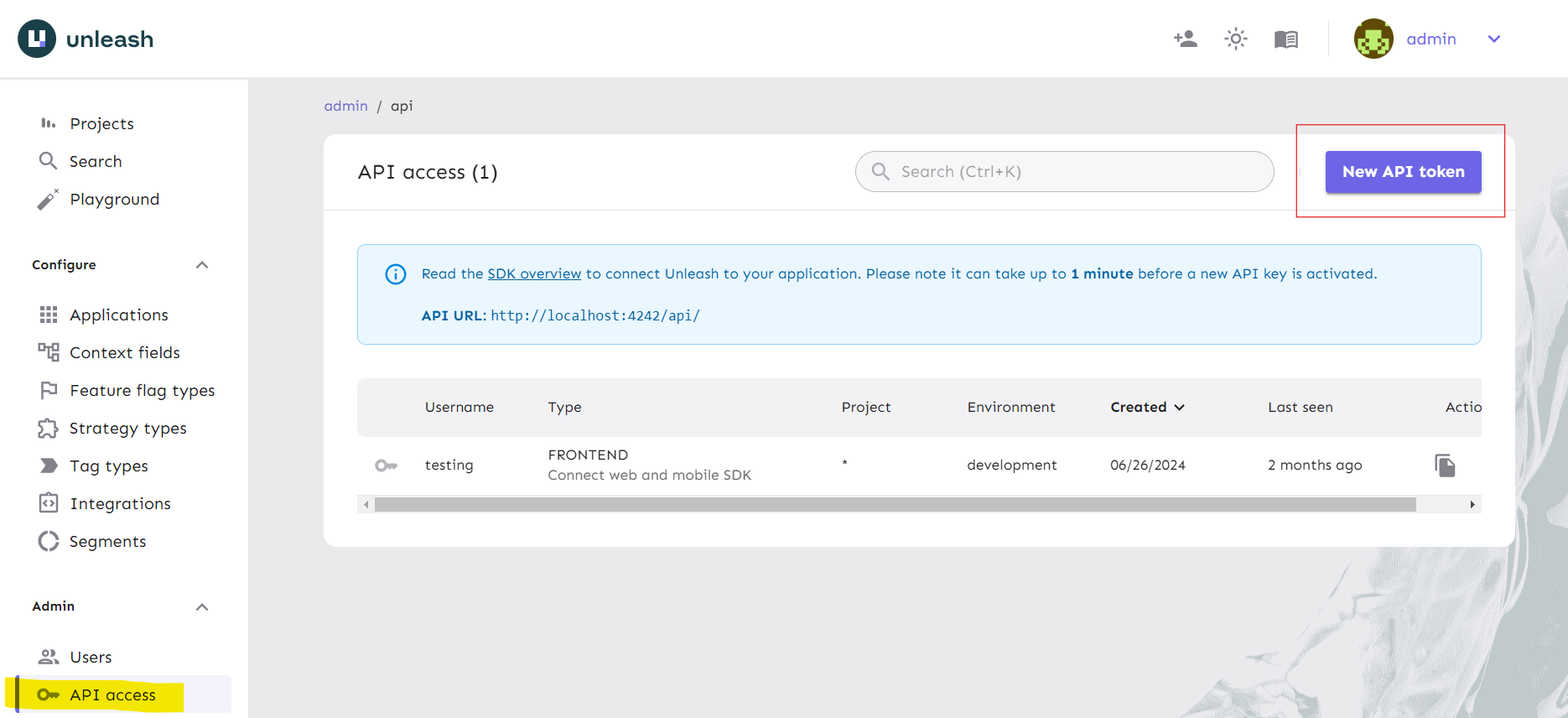
Provide a name for the token and choose the SDK type. Since this blog focuses on React, select the Client-side SDK. After choosing the environment, click “Create Token.” Be sure to save the token for future reference.
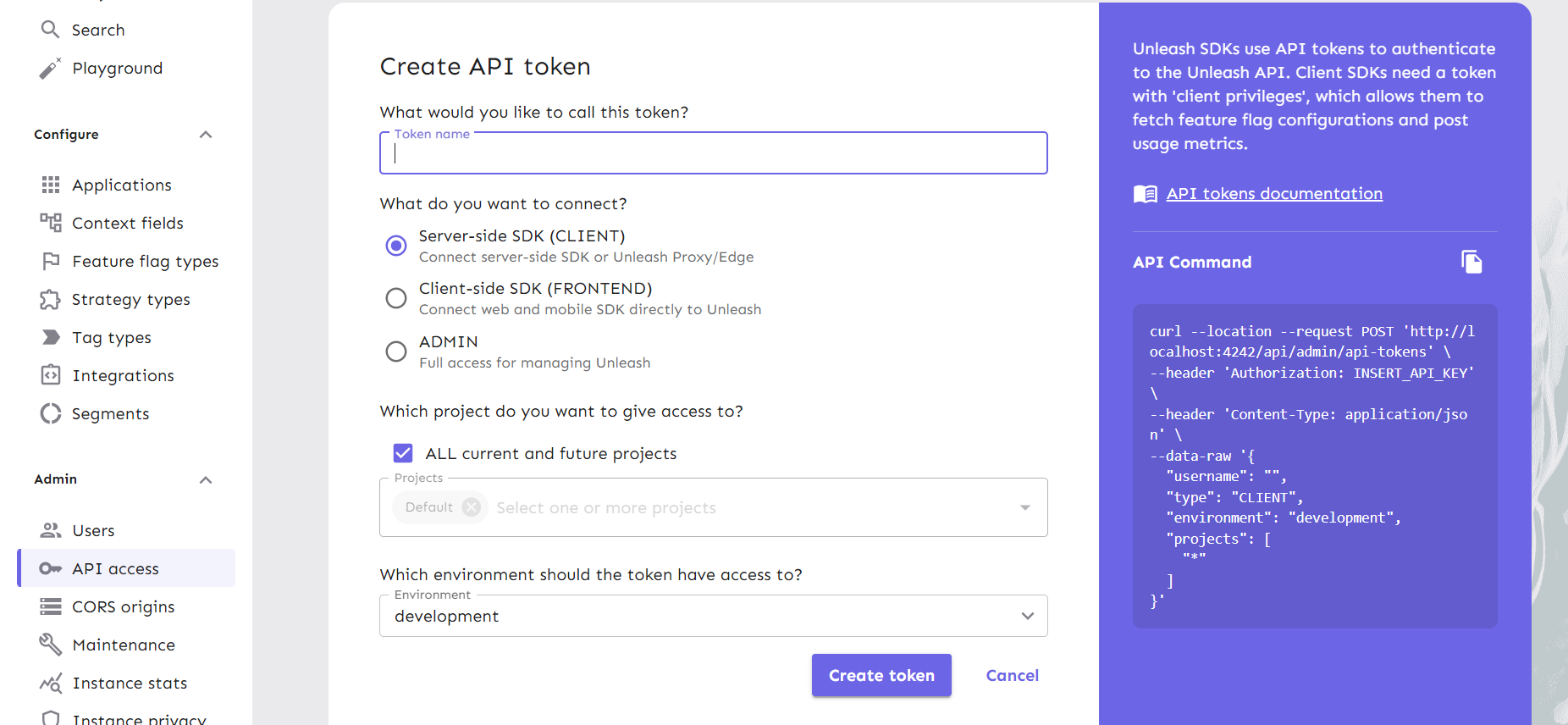
Unleash React SDK
With your token created, you’re set to integrate Unleash into your React application. Start by installing and configuring the client-side SDK to align with your server’s setup.
Installing package
Use either of the commands (as per your environment) to install the sdk.
npm install @unleash/proxy-client-react unleash-proxy-client
# or
yarn add @unleash/proxy-client-react unleash-proxy-client
Configuration
Now import the sdk, configure and wrap your <App/> as following (typically in index.js/ts):
import { createRoot } from 'react-dom/client';
import { FlagProvider } from '@unleash/proxy-client-react';
const config = {
url: '<unleash-url>/api/frontend',
clientKey: '<your-token>',
refreshInterval: 15,
appName: 'your-app-name',
};
const root = createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<FlagProvider config={config}>
<App />
</FlagProvider>
</React.StrictMode>
);
Replace <unleash-url> with Unleash server url, in our case it’s http://localhost:4242 . Replace <your-token> with the token that we created in step 2 of installation. Replace your-app-name with your App’s name (it will be only used to identify your application).
Creating Feature-flag
On the dashboard (in projects tab) click on the default project. You’ll see a window like:
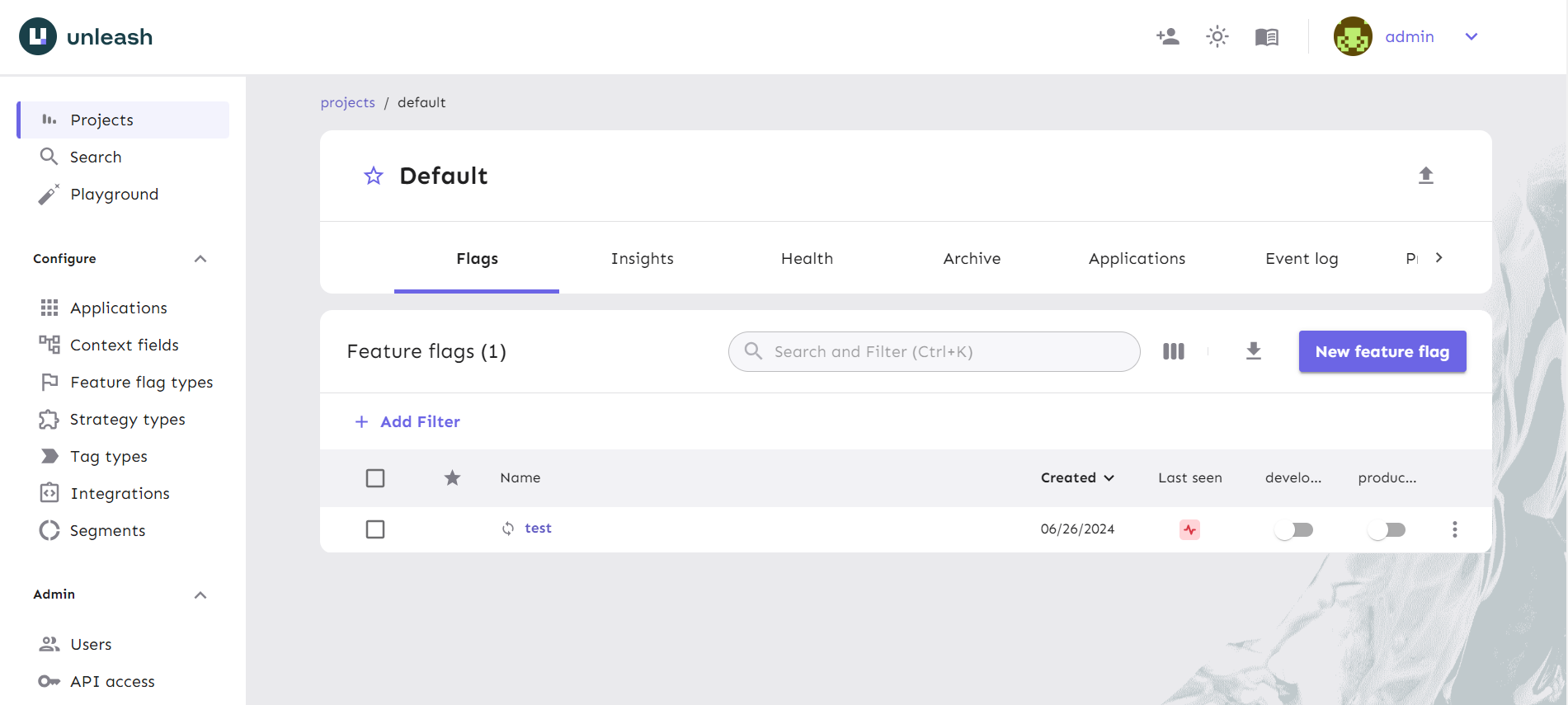
P.s. You might not see the “test” flag as I created it earlier.
Click on the “New feature flag” button. You will see a window like this:
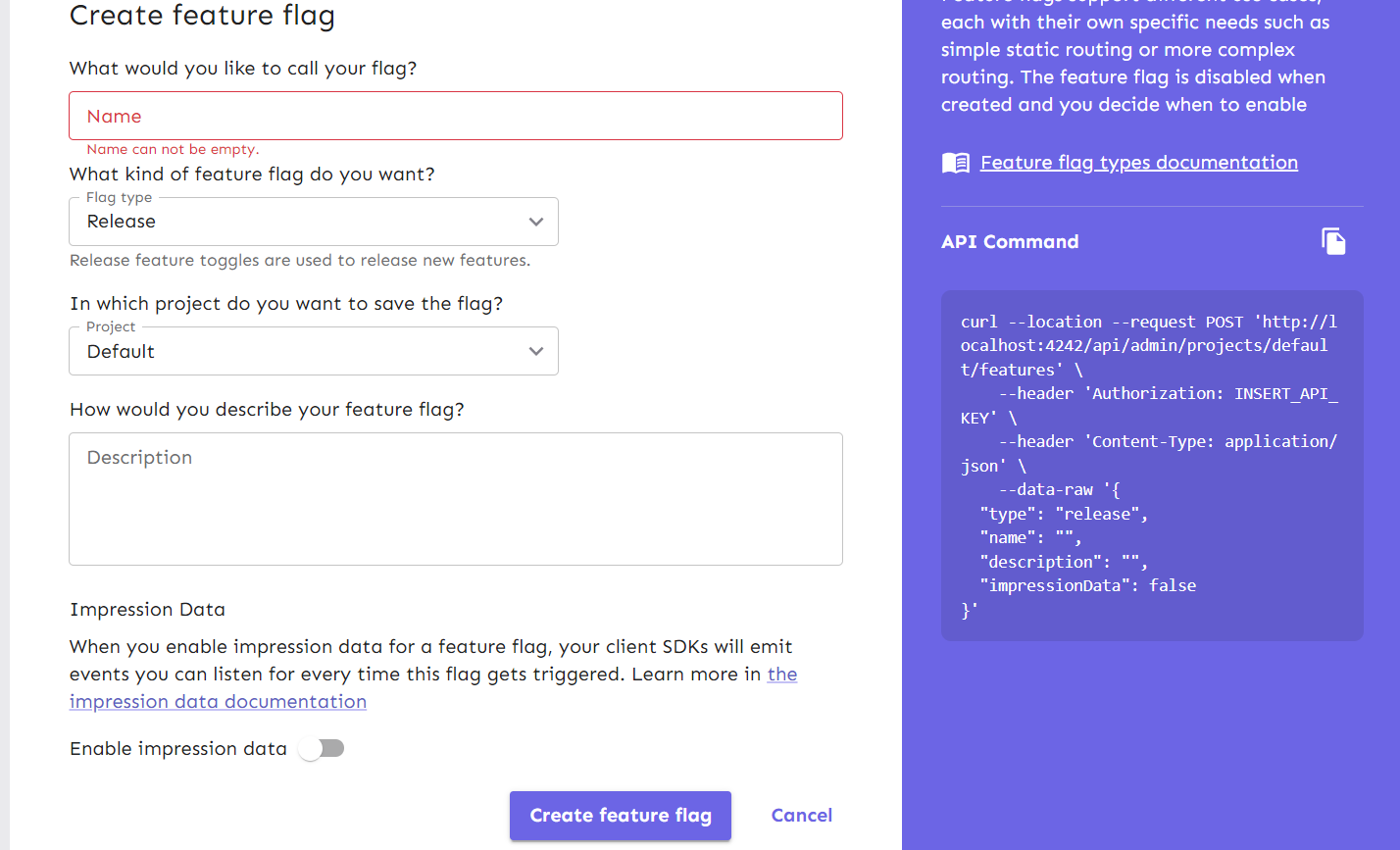
Name your token, let the flag type and project remain the same. Toggle-on “Enable Impression data” and click on “Create feature flag”.
Congratulations! You have created your first feature flag. Now use it in your application.
Consuming feature flag
Head to your component/feature which you want to bind with the feature flag. And bind it as following:
import { useFlag } from '@unleash/proxy-client-react';
const TestComponent = () => {
const enabled = useFlag('travel.landing');
if (enabled) {
return <SomeComponent />;
}
return <AnotherComponent />;
};
export default TestComponent;
Now your Test component is bound with the feature flag. You can toggle your flag from the Unleash GUI (click the toggle button under development/production) and see the difference in your frontend:
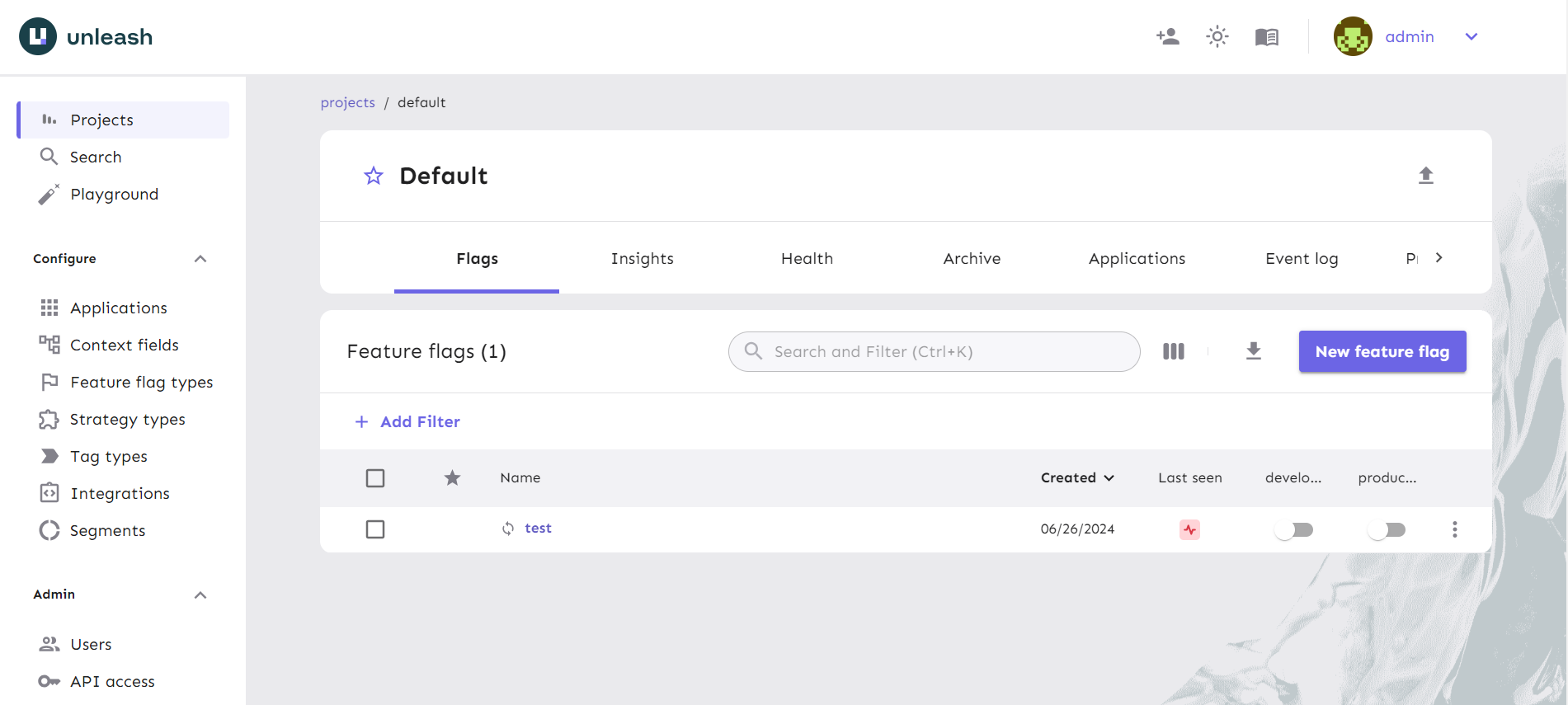
Conclusion
Integrating feature flags into your React applications with the Unleash SDK provides significant benefits, including enhanced user experience through A/B testing and reduced risk with incremental rollouts. By utilizing feature flags, you can make your development process more adaptable and responsive to user feedback, allowing for real-time feature adjustments without the need for frequent redeployments. Unleash’s powerful integration features make it an essential tool for development teams aiming to implement feature flags smoothly and efficiently.
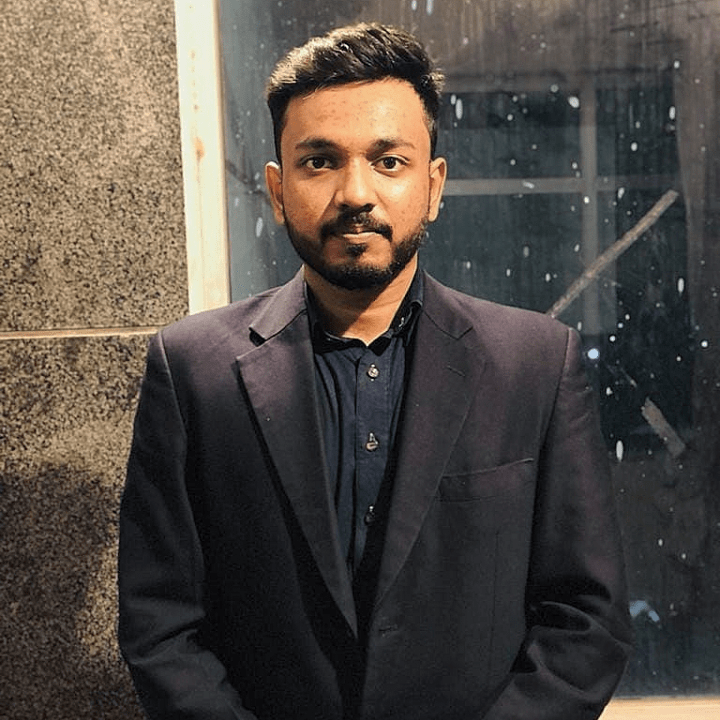